This 2 step tutorial will explain how to register in Auth0 and setup User Login for SPA application. The tutorial explains the steps in React but can be substituted with Angular, Vue or any Javascript framework
Step 1: Auth0 registration
Step 2: User login setup using React
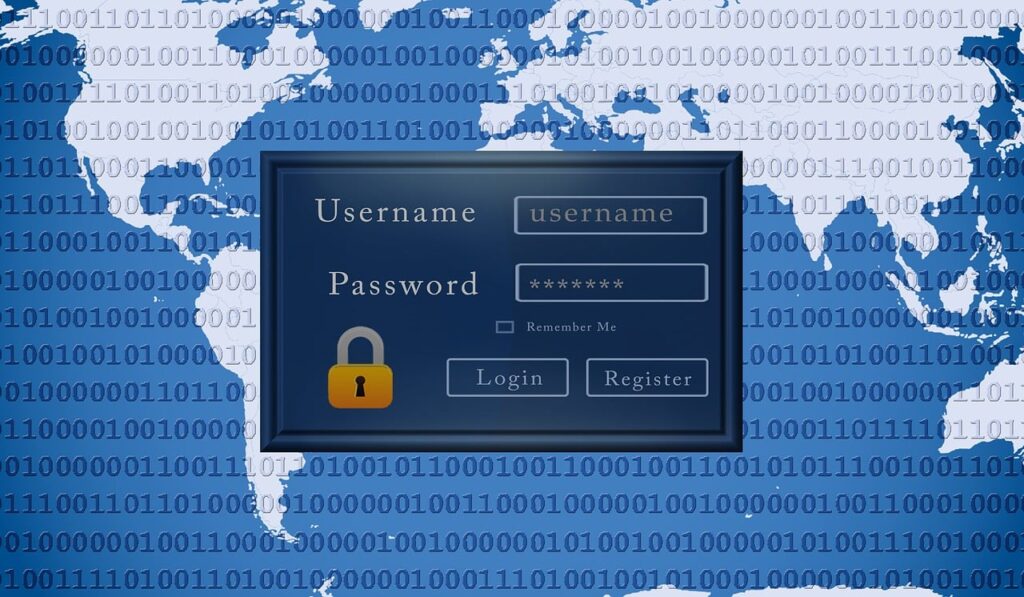
Step 2 of the tutorial will use the Auth0 SPA app created in the previous step.
Create a sample react application and install the auth0 library
npm install @auth0/auth0-react
Create a .env file and store the client id and domain from the Auth0 application got in the previous steps
REACT_APP_AUTH0_DOMAIN = dev-abc.us.auth0.com
REACT_APP_AUTH0_CLIENT_ID = 555ABC
Modify the index.js to enclose the app within the Auth0Provider
import { Auth0Provider } from '@auth0/auth0-react';
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
const domain = process.env.REACT_APP_AUTH0_DOMAIN;//from the .env file
const clientId = process.env.REACT_APP_AUTH0_CLIENT_ID;//from the .env file
ReactDOM.render(
<React.StrictMode>
<Auth0Provider
domain={domain}
clientId={clientId}
redirectUri={window.location.origin}
>
<App />
</Auth0Provider>
</React.StrictMode>,
document.getElementById('root')
);
Create a component called Login in the src/components folder for the sign in page. Import useAuth0 hook from the auth0-react library. We are going to use the loginWithRedirect to redirect the login to Auth0 page and isAuthenticated to verify if the user is authenticated from the useAuth0 hook
The Sign In button will be visible only if the user is not authenticated. When the Sign In button is clicked, the user will be redirected to the Auth0 sign in page
import { useAuth0 } from '@auth0/auth0-react';
const Login = () => {
const { loginWithRedirect, isAuthenticated } = useAuth0();
return (
!isAuthenticated && (
<button onClick={() => loginWithRedirect()}>
Sign In
</button>
)
)
}
export default Login
Modify the App.js file to include the Login component
import Login from "./components/Login";
function App() {
return (
<main className="column">
<h1>React App Login</h1>
<Login />
</main>
);
}
export default App;
That is all needed to setup the user sign in using React and Auth0. Now start the application using npm start and the login page will be displayed
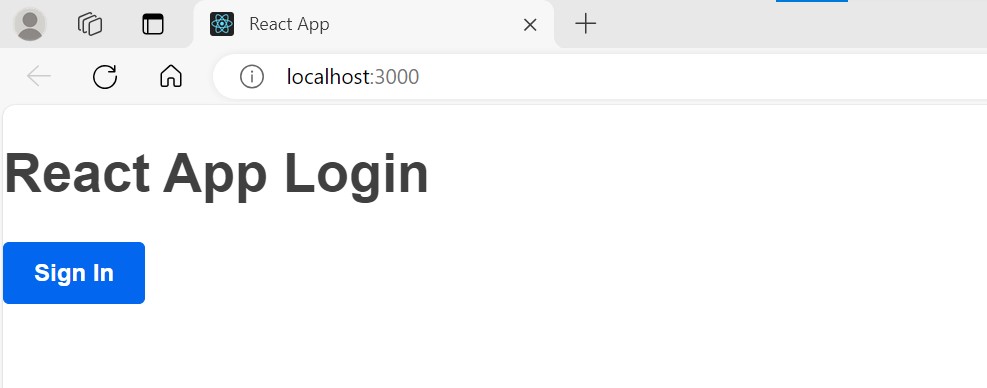
Click on Sign In and the user will be redirected to the sign in page. Since we have configured the Auth0 application to use Google as sign in option, we can see the Continue with Google link
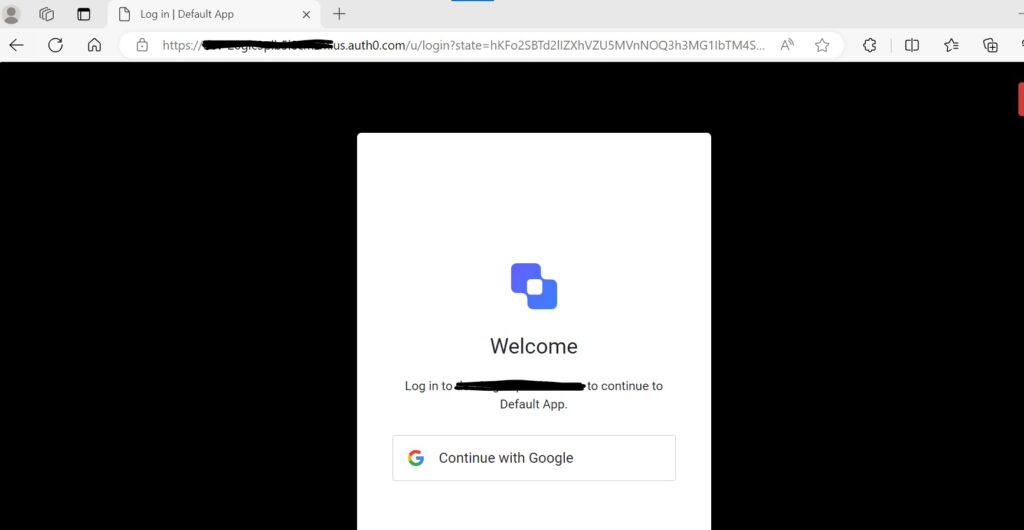
Hope you enjoyed the tutorial. Please keep reading for more on Auth0 and other latest technologies