This post will walk you through the steps to create a simple Docker node application which has cron jobs scheduled to run on Argo Worflows. It is completely serverless architecture
Create a simple node application with package.json
{
"name": "argonodejobs",
"version": "1.0.0",
"description": "",
"main": "app.js",
"scripts": {
"start": "node app.js",
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC",
"dependencies": {
"request": "^2.81.0"
}
}
Create a Docker file. We will use node v16 as runtime environment
FROM node:16-alpine
USER root
COPY package*.json ./
RUN npm install
COPY . .
Argo workflows need Kubernetes cluster and kubectl setup to access the cluster. There are multiple options to setup the Kubernetes cluster locally. This tutorial users Docker Desktop. Enable Kubernetes by clicking on Settings -> Kubernetes
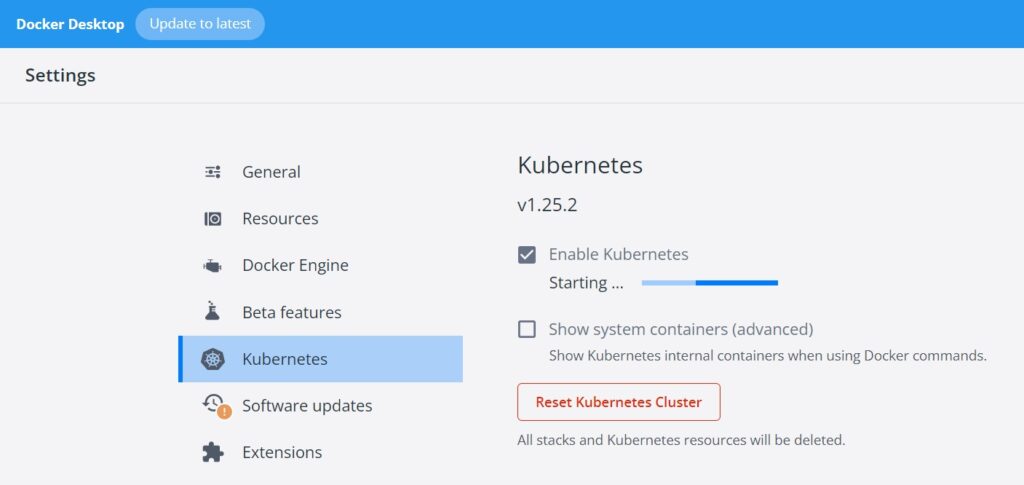
Install Argo Workflows using the following commands. The download versions are found in argo workflows github https://github.com/argoproj/argo-workflows/releases/
kubectl create ns argo
kubectl apply -n argo -f https://github.com/argoproj/argo-workflows/releases/download/v3.4.5/install.yaml
Open a port forward so you can access the UI.This will serve the UI on https://localhost:2746.
kubectl -n argo port-forward svc/argo-server 2746:2746
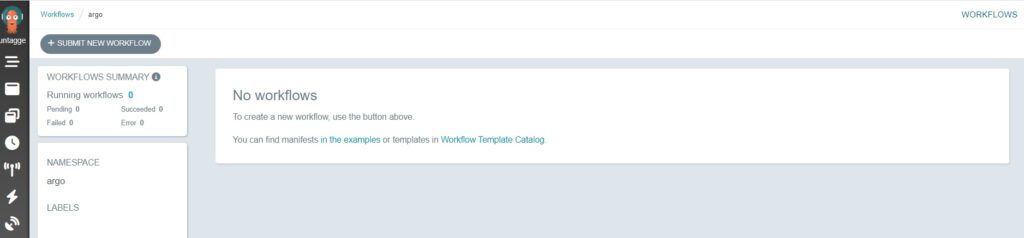
Create a javascript file test.js on the root folder with just a simple function which prints test
(function () {
printtest();
})();
function printtest() {
console.log("testing")
}
Test locally by building and running the docker image to ensure there are no errors with the docker application
docker build -t argotest .
docker run argotest node test.js
Create a DockerHub account. Publish the docker image to DockerHub repository
docker login -u <dockerhubaccountname>
docker build -t argotest .
docker image tag argotest <dockerhubaccountname>/argotest:latest
docker image push <dockerhubaccountname>/argotest:latest
Create a argo cron workflow test.yml file to run the test.js file on a cron schedule
metadata:
generateName: argotest-cronjobs-test-
spec:
workflowMetadata:
schedule: 35 12 * * *
timezone: "America/Los_Angeles"
workflowSpec:
entrypoint: argotest
templates:
- name: argotest
container:
image: docker.io/<dockerhubaccountname>/argotest
command: [node]
args: ["test.js"]
ttlStrategy:
secondsAfterCompletion: 300
podGC:
strategy: OnPodCompletion
Use either the argo submit command or the UI to create the new workflow. This tutorial will use the UI to create the new workflow. Navigate to the Cron Workflows tab in the left menu
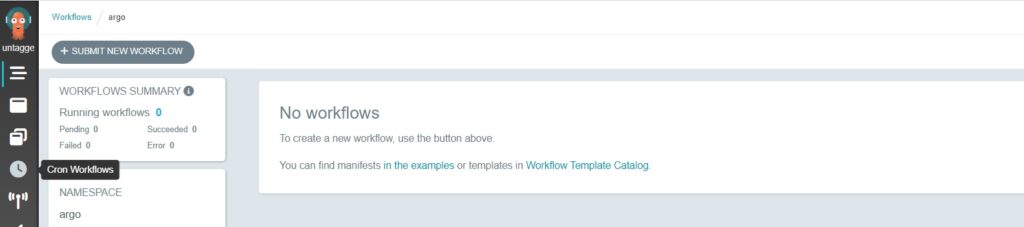
Click on CREATE NEW CRON WORKFLOW. Use the UPLOAD FILE option to upload the test.yml file created in the previous step.
A new CRON WORKFLOW will be created.